MyBatis 多表操作教程
1.1 一对一查询
1.1.1 概述
关系数据库中第一个表中的单个行只可以与第二个表中的一个行相关,且第二个表中的一个行也只可以与第一个表中的一个行相关。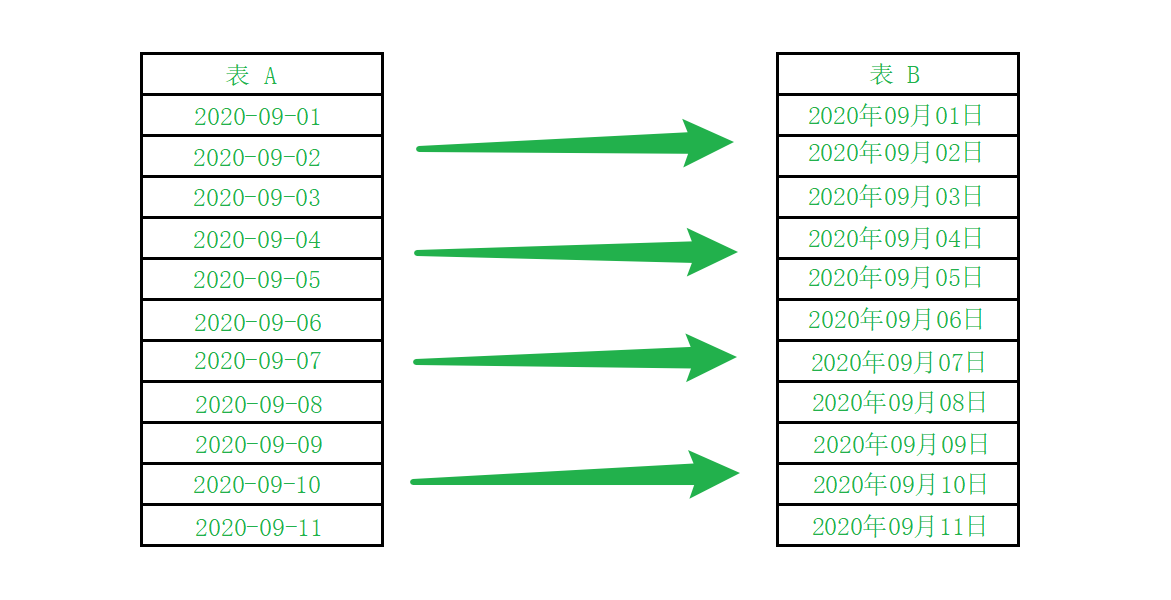
1.1.2 创建实体类
public class Student {
private Integer id;
private String name;
private Boolean age;
private String sex;
private StudentStatus studentStatus;
}
public class StudentStatus {
private Integer id;
private String num;
private String major;
}
1.1.3 创建 DAO 接口
public interface StudentDao {
public List<Student> findAll();
}
1.1.4 结果映射
resultMap 元素是 MyBatis 中最重要最强大的元素。它可以从 90% 的 JDBC ResultSets 数据提取代码中解放出来,并在一些情形下允许进行一些 JDBC 不支持的操作。实际上,在为一些比如连接的复杂语句编写映射代码的时候,一份 resultMap 能够代替实现同等功能的长达数千行的代码。resultMap 的设计思想是,对于简单的语句根本不需要配置显式的结果映射,而对于复杂一点的语句只需要描述它们的关系就行了。之前已经使用过简单映射语句了,但并没有显式指定 resultMap。只是简单的使用 resultType 将所有的列映射到对象的属性上,需要注意的是列名与属性名一致才能映射,解决列名不匹配还是需要使用 resultMap。
<resultMap id="userResultMap" type="User">
<result property="id" column="user_id" />
<result property="username" column="user_name"/>
<result property="password" column="hashed_password"/>
</resultMap>
<select id="selectUsers" resultMap="userResultMap">
select user_id, user_name, hashed_password from some_table where id = #{id}
</select>
1.1.5 配置 mapper
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.software.mybatis.dao.StudentDao">
<resultMap id="resMap" type="student">
<result property="studentStatus.id" column="st_id"/>
<result property="studentStatus.major" column="major"/>
<result property="studentStatus.num" column="num"/>
</resultMap>
<select id="findAll" resultMap="resMap">
select * from student s, student_status st where s.st_id = st.st_id
</select>
</mapper>
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
上面这种配置会将自动将列名一致的映射到 type 指定的实体类中,该实体类中属性类型为对象的则需要单独拿出来映射。还可以使用 association 进行复杂的映射,我们发现未配置的属性无法进行映射。产生这个问题的原因是 resultMap 的自动映射未打开,使用 autoMapping 设置这个属性为 true/false,MyBatis 将会为本结果映射开启/关闭自动映射。
<mapper namespace="com.software.mybatis.dao.StudentDao">
<resultMap id="resMap" type="com.software.mybatis.entity.Student">
<result property="id" column="id"/>
<result property="name" column="name"/>
<result property="sex" column="sex"/>
<result property="age" column="age"/>
<association property="studentStatus" javaType="com.software.mybatis.entity.StudentStatus">
<result property="id" column="st_id"/>
<result property="major" column="major"/>
<result property="num" column="num"/>
</association>
</resultMap>
<select id="findAll" resultMap="resMap">
select * from student s, student_status st where s.st_id = st.st_id
</select>
</mapper>
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.software.mybatis.dao.StudentDao">
<resultMap id="resMap" type="student" autoMapping="true">
<association property="studentStatus" resultMap="stMap" />
</resultMap>
<resultMap id="stMap" type="StudentStatus" autoMapping="true"/>
<select id="findAll" resultMap="resMap">
select * from student s, student_status st where s.st_id = st.st_id
</select>
</mapper>
1.1.6 核心配置
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
<typeAliases>
<package name="com.software.mybatis.entity"/>
</typeAliases>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/db"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="student-mapper.xml"/>
</mappers>
</configuration>
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
-
18
-
19
-
20
-
21
-
22
-
23
-
24
-
25
-
26
-
27
-
28
1.1.7 测试
public class MybatisDemo {
@Test
public void TestA() throws IOException {
InputStream resourceAsStream = Resources.getResourceAsStream("mybatis.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);
SqlSession sqlSession = sqlSessionFactory.openSession();
List<Student> list = sqlSession.selectList("com.software.mybatis.dao.StudentDao.findAll");
System.out.println(list);
}
}
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
-
18
-
19
-
20
-
21
-
22
-
23
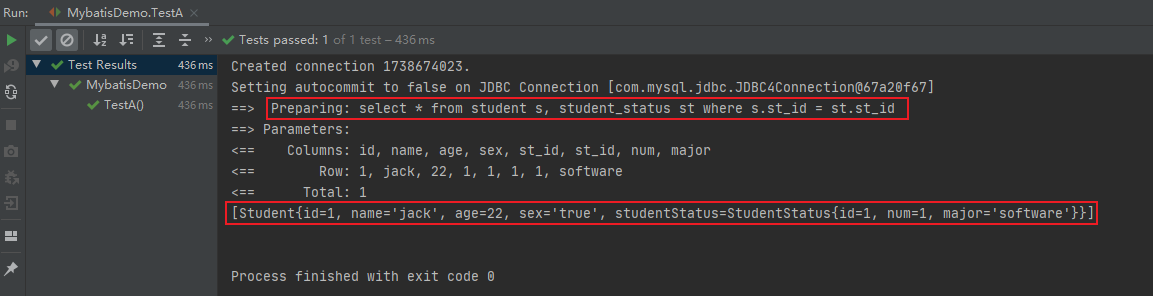
1.2 一对多查询
1.2.1 概述
一对多关系是关系数据库中两个表之间的一种关系,该关系中第一个表中的单个行可以与第二个表中的一个或多个行相关,但第二个表中的一个行只可以与第一个表中的一个行相关。
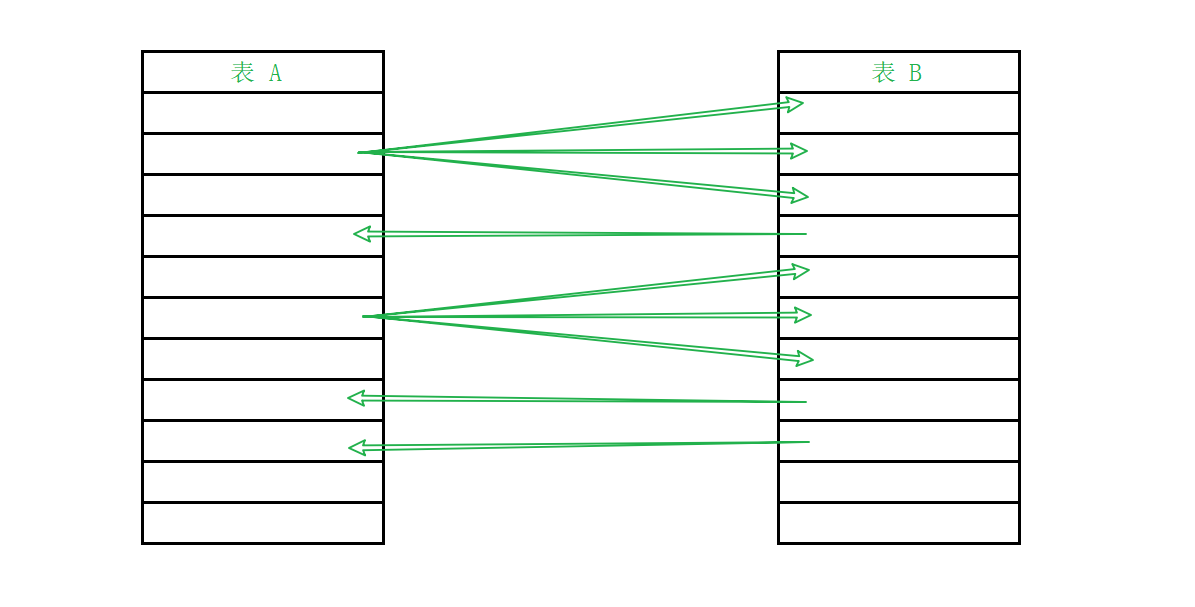
1.2.2 创建实体类
public class Student {
private Integer sId;
private String sName;
private Long sAge;
private String sSex;
private Integer cId;
}
public class Class {
private Integer cId;
private String cName;
private String cAddr;
private List<Student> students;
}
1.1.3 创建 DAO 接口
public interface ClassDao {
public List<Class> findAll();
}
1.1.4 配置 mapper
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.software.mybatis.dao.ClassDao">
<resultMap id="resMap" type="Class">
<result property="cId" column="c_id"/>
<result property="cName" column="c_name"/>
<result property="cAddr" column="c_addr"/>
<collection property="students" ofType="Student">
<result property="sId" column="s_id" />
<result property="sName" column="s_name"/>
<result property="sAge" column="s_age"/>
<result property="sSex" column="s_sex"/>
<result property="cId" column="c_id"/>
</collection>
</resultMap>
<select id="findAll" resultMap="resMap">
select * from student s, class c where s.c_id = c.c_id
</select>
</mapper>
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
-
18
-
19
1.1.5 测试
public class MybatisDemo {
@Test
public void TestA() throws IOException {
InputStream resourceAsStream = Resources.getResourceAsStream("mybatis.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);
SqlSession sqlSession = sqlSessionFactory.openSession();
List<Class> list = sqlSession.selectList("com.software.mybatis.dao.ClassDao.findAll");
for (Class aClass : list) {
System.out.println(aClass);
}
}
}
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
-
18
-
19
-
20
-
21
-
22
-
23
-
24
-
25
-
26
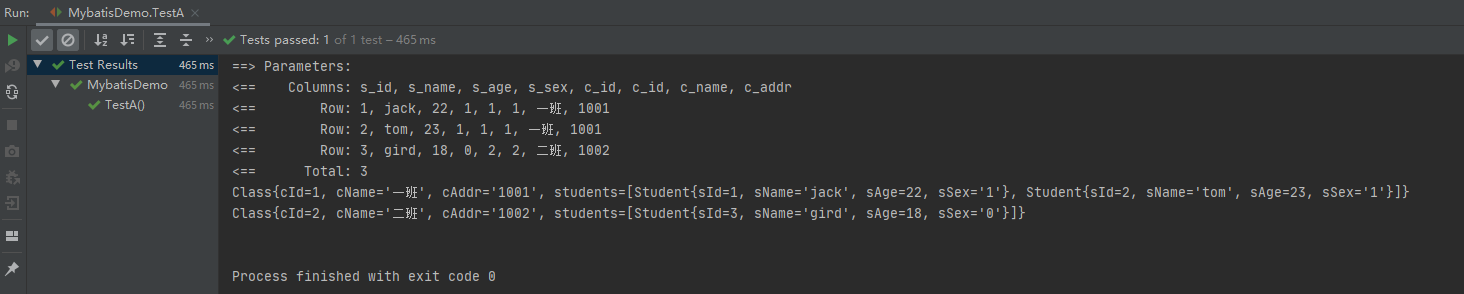
1.3 多对多查询
1.3.1 概述
多对多关系是关系数据库中两个表之间的一种关系, 该关系中第一个表中的一个行可以与第二个表中的一个或多个行相关。第二个表中的一个行也可以与第一个表中的一个或多个行相关。该关系一般会借助第三方表实现。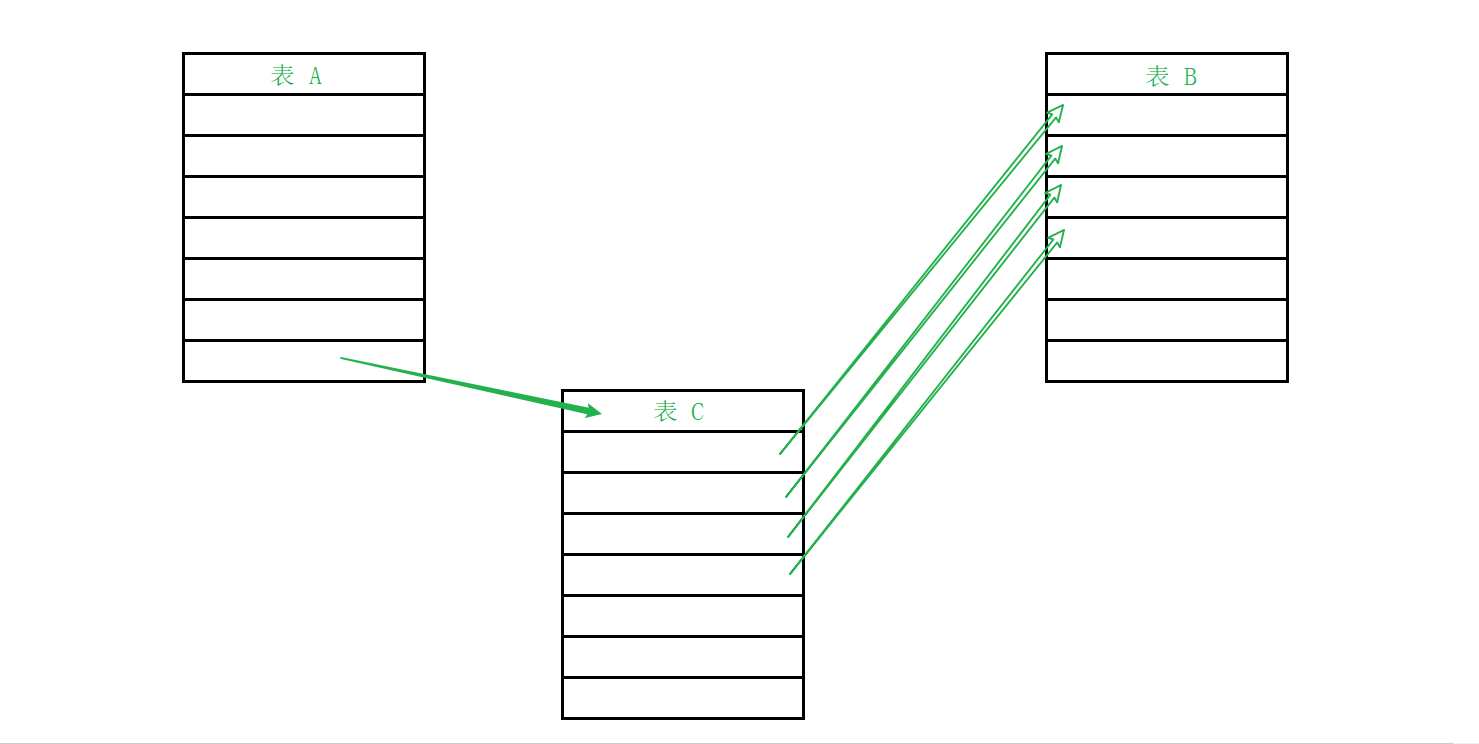
1.3.2 创建实体类
public class Course {
private Integer cId;
private String cName;
private List<Student> students;
}
public class Student {
private Integer sId;
private String sName;
private Long sAge;
private String sSex;
private List<Course> courses;
}
1.3.3 创建 DAO 接口
public interface CourseDao {
public List<Course> findAll();
}
public interface StudentDao {
public List<Student> findAll();
}
1.3.4 配置 mapper
☞ student-mapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.software.mybatis.dao.StudentDao">
<resultMap id="resMap" type="Student">
<result property="sId" column="s_id" />
<result property="sName" column="s_name"/>
<result property="sAge" column="s_age"/>
<result property="sSex" column="s_sex"/>
<collection property="courses" ofType="Course">
<result property="cId" column="c_id"/>
<result property="cName" column="c_name"/>
</collection>
</resultMap>
<select id="findAll" resultMap="resMap">
select * from course c, student s, s_c sc where c.c_id = sc.c_id and s.s_id = sc.s_id
</select>
</mapper>
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
☞ course-mapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.software.mybatis.dao.CourseDao">
<resultMap id="resMap" type="Course">
<result property="cId" column="c_id"/>
<result property="cName" column="c_name"/>
<collection property="students" ofType="Student">
<result property="sId" column="s_id" />
<result property="sName" column="s_name"/>
<result property="sAge" column="s_age"/>
<result property="sSex" column="s_sex"/>
</collection>
</resultMap>
<select id="findAll" resultMap="resMap">
select * from course c, student s, s_c sc where c.c_id = sc.c_id and s.s_id = sc.s_id
</select>
</mapper>
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
1.3.5 测试
public class MybatisDemo {
@Test
public void TestA() throws IOException {
InputStream resourceAsStream = Resources.getResourceAsStream("mybatis.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);
SqlSession sqlSession = sqlSessionFactory.openSession();
List<Course> courseList = sqlSession.selectList("com.software.mybatis.dao.CourseDao.findAll");
List<Student> studentList = sqlSession.selectList("com.software.mybatis.dao.StudentDao.findAll");
System.out.println("### 课程 ###");
for (Course course : courseList) {
System.out.println(course);
}
System.out.println("### 学生 ###");
for (Student student : studentList) {
System.out.println(student);
}
}
}
-
1
-
2
-
3
-
4
-
5
-
6
-
7
-
8
-
9
-
10
-
11
-
12
-
13
-
14
-
15
-
16
-
17
-
18
-
19
-
20
-
21
-
22
-
23
-
24
-
25
-
26
-
27
-
28
-
29
-
30
-
31
-
32
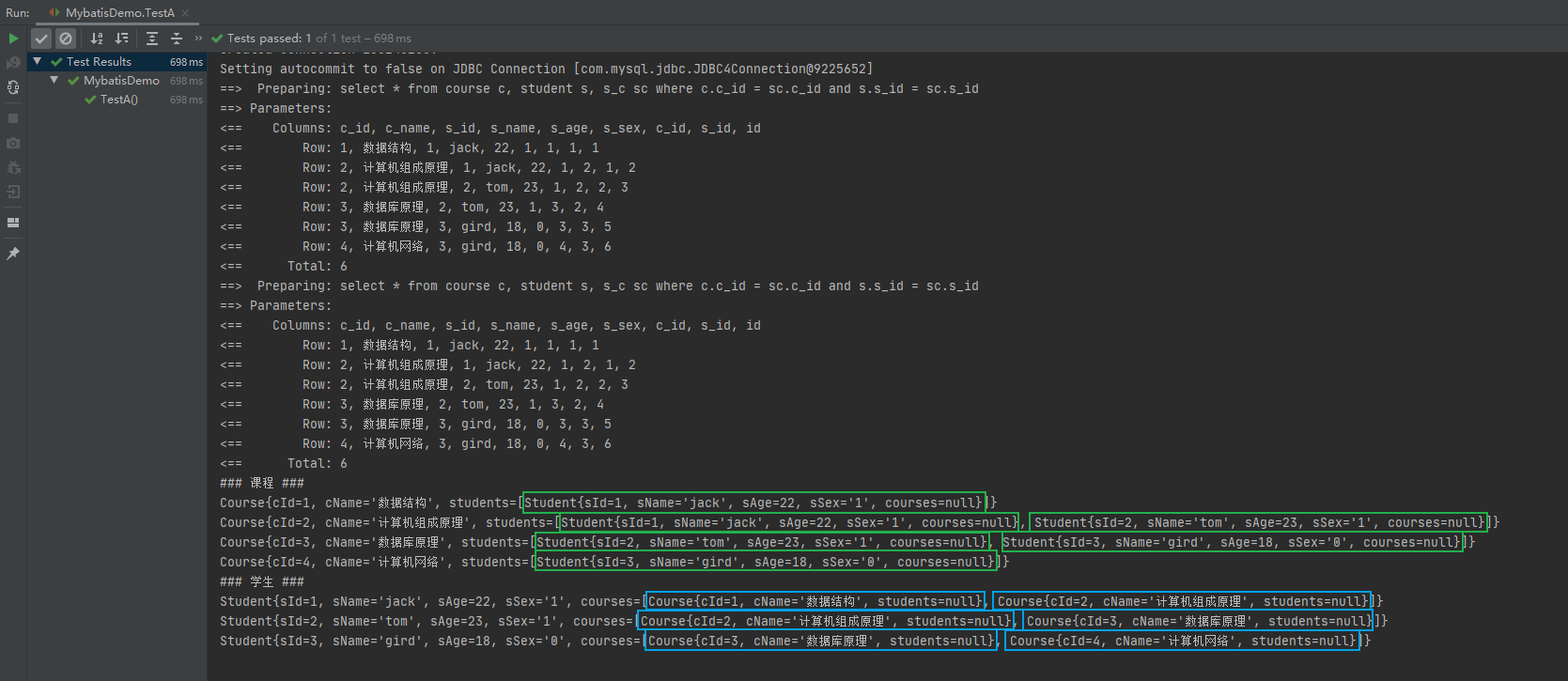